Setup NGX-Translate with Angular 19 (Standalone)
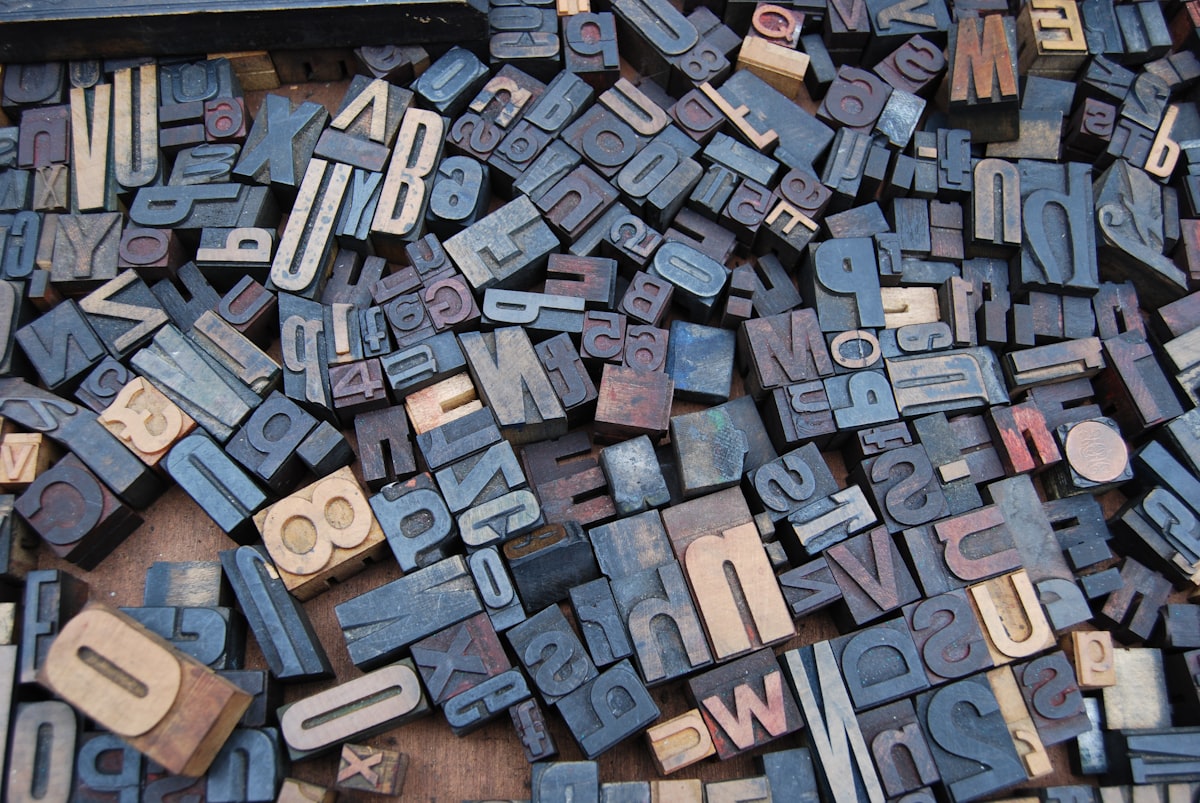
When I first started working with Angular 19 standalone components, I quickly realized how challenging it was to find a clear and up-to-date guide for integrating ngx-translate. Most resources were outdated or didn’t cater to the standalone approach introduced in recent Angular versions. After piecing together bits of information and experimenting with my own setup, I finally got it working—and now I want to share that process with you. If you’re struggling to integrate ngx-translate into your Angular 19 standalone project, this guide is here to save you time and frustration!
Pre requirements
- Install ngx-translate
npm i @ngx-translate/core
- Install http loader to dynamically load the necessary translation file
npm i @ngx-translate/http-loader
Configuration
To get started with ngx-translate
in an Angular 19 standalone project, you need to configure the translation loader and set up the global providers. This ensures that the translation files are loaded correctly and the default language is set. Below is the configuration using TranslateHttpLoader
to load JSON files from an i18n/
folder.
Translation files
Create a i18n
folder in your public
folder. In this folder you can create your translation files, like en.json
ord de.json
It can look like this:
{
"hello": "Hello, Welcome"
}
Global config
The global configuration defines how translations are loaded and sets up the default language for your application. Using ApplicationConfig
, we provide the necessary services for ngx-translate
, including a factory function for TranslateHttpLoader
. This factory specifies where your translation files are stored (i18n/
) and their file extension (.json
).
import { ApplicationConfig } from '@angular/core';
import { provideHttpClient, withInterceptorsFromDi } from '@angular/common/http';
import { provideTranslateService, TranslateLoader } from '@ngx-translate/core';
import { TranslateHttpLoader } from '@ngx-translate/http-loader';
import { HttpClient } from '@angular/common/http';
// Factory function for the TranslateHttpLoader
export function httpLoaderFactory(http: HttpClient) {
return new TranslateHttpLoader(http, 'i18n/', '.json');
}
// Global application configuration
export const appConfig: ApplicationConfig = {
providers: [
provideHttpClient(withInterceptorsFromDi()),
provideTranslateService({
loader: {
provide: TranslateLoader,
useFactory: httpLoaderFactory,
deps: [HttpClient],
},
defaultLanguage: 'en', // Set the default language
}),
],
};
Import in standalone component
In standalone components, you need to import TranslateModule
to make translation functionality available in your templates. Additionally, inject TranslateService
into the component's constructor to manage translations programmatically.
import { Component } from '@angular/core';
import { TranslateModule, TranslateService } from '@ngx-translate/core';
@Component({
selector: 'app-root',
imports: [TranslateModule],
templateUrl: './app.component.html',
styleUrl: './app.component.scss',
standalone: true,
})
export class AppComponent {
constructor(private translate: TranslateService) {}
}
Automatic browser language detection
To enhance user experience, you can automatically detect the user's browser language and set it as the default if it's included in your supported languages. The following code checks the browser's language, matches it with your supported languages (en
, de
), and sets it as the active language. If no match is found, it defaults to English (en
).
constructor(private translate: TranslateService) {
// Set included languages
this.translate.addLangs(['en', 'de']);
const browserLang = navigator.languages
? navigator.languages[0].split('-')[0]
: navigator.language.split('-')[0];
// Get the current browser language, if included set it
const defaultLang = this.translate.getLangs().includes(browserLang) ? browserLang : 'en';
// Set the default and current language
this.translate.setDefaultLang(defaultLang);
}
switchLanguage(lang: string) {
this.translate.use(lang);
}
You can also switch languages dynamically using a simple method:
switchLanguage(lang: string) {
this.translate.use(lang);
}
Usage
Once everything is set up, using translations in your templates is straightforward. Simply use the translate
pipe with your translation keys as shown below:
<p>{{ "your-key" | translate }}</p>
With this setup, you can efficiently manage translations in your Angular 19 standalone project!